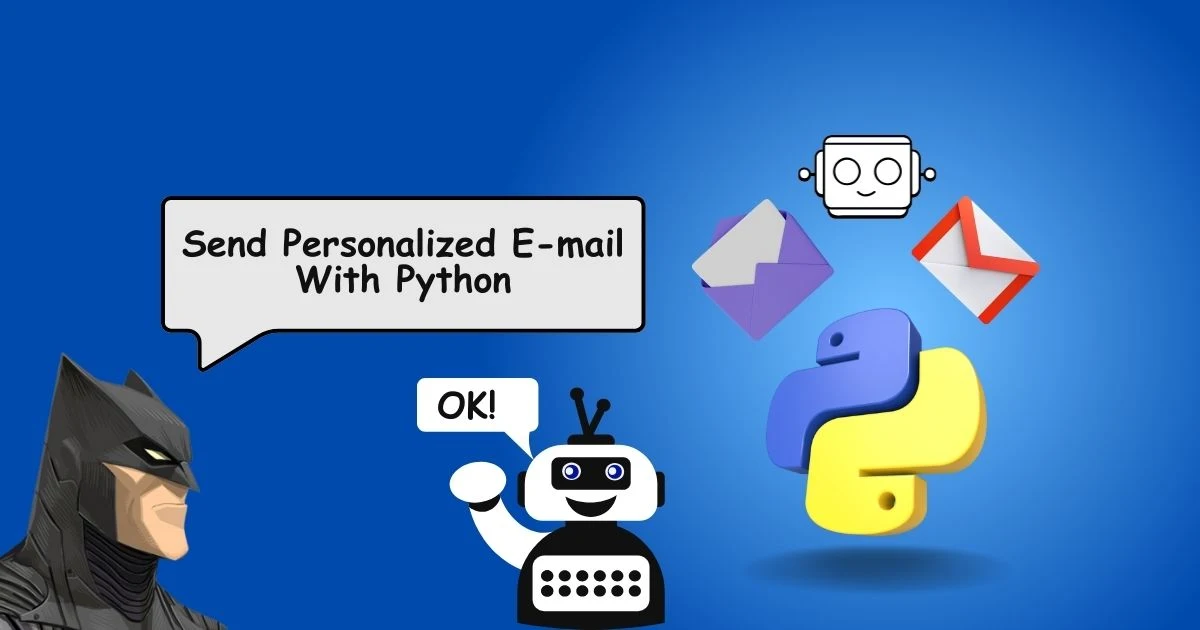
Welcome, Python enthusiasts! In this tutorial, we'll explore the fascinating world of sending personalized emails using Python. Whether you are a beginner or an experienced developer, this guide will help you create customized and engaging email content that stands out in your recipients' inboxes.
Getting Started
Before diving into the code, let's ensure you have the necessary tools installed:
pip install smtplib email
We'll be using the built-in smtplib
and email
libraries to handle the email functionality. Once you have these installed, you're ready to proceed.
Setting Up Your Email Account
For this tutorial, we'll use a Gmail account to send emails. Make sure to enable "Less secure app access" in your Google account security settings. Additionally, generate an "App Password" to use in your Python script.
Writing the Python Script
Let's create a simple Python script that sends a personalized email. We'll use placeholders for the recipient's name and customize the email subject and body accordingly.
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
def send_personalized_email(to_email, recipient_name):
# Email configuration
sender_email = "your@gmail.com"
app_password = "your_app_password"
subject = f"Hello, {recipient_name}! Personalized Greetings from Python"
# Email body
body = f"Dear {recipient_name},\n\n"\
"I hope this email finds you well. "\
"This is a personalized greeting sent using Python. "\
"Feel free to customize this message further.\n\n"\
"Best regards,\nYour Name"
# Create the email
message = MIMEMultipart()
message["From"] = sender_email
message["To"] = to_email
message["Subject"] = subject
# Attach the body to the email
message.attach(MIMEText(body, "plain"))
# Connect to the SMTP server
with smtplib.SMTP("smtp.gmail.com", 587) as server:
server.starttls()
server.login(sender_email, app_password)
# Send the email
server.sendmail(sender_email, to_email, message.as_string())
# Example usage
recipient_email = "recipient@example.com"
recipient_name = "John"
send_personalized_email(recipient_email, recipient_name)
Replace your@gmail.com
with your Gmail address and your_app_password
with the generated app password. Also, customize the email body as needed.
Adding More Personalization
Now that you've sent a basic personalized email, let's take it a step further. Imagine you have a list of recipients with their names and email addresses stored in a CSV file. We can modify the script to read this file and send personalized emails to each recipient.
import csv
def send_personalized_emails_from_csv(csv_file):
with open(csv_file, mode="r") as file:
reader = csv.DictReader(file)
for row in reader:
send_personalized_email(row["email"], row["name"])
# Example usage
csv_file_path = "recipients.csv"
send_personalized_emails_from_csv(csv_file_path)
Create a CSV file named recipients.csv
with columns email
and name
. Populate it with your recipient's information, and the script will automatically send personalized emails to each one.
Handling Attachments
Want to send attachments along with your personalized email? No problem! Let's enhance our script to include this functionality:
from email.mime.base import MIMEBase
from email import encoders
def send_personalized_email_with_attachment(to_email, recipient_name, attachment_path):
# ... (previous code remains unchanged)
# Attach the body to the email
message.attach(MIMEText(body, "plain"))
# Attach the file
attachment = open(attachment_path, "rb")
base = MIMEBase("application", "octet-stream")
base.set_payload((attachment).read())
encoders.encode_base64(base)
base.add_header("Content-Disposition", f"attachment; filename= {attachment_path}")
message.attach(base)
# Connect to the SMTP server
with smtplib.SMTP("smtp.gmail.com", 587) as server:
# ... (previous code remains unchanged)
Update the send_personalized_email
function to include an additional attachment_path
parameter. Now you can send personalized emails with attachments by providing the file path when calling the function.
Conclusion
Congratulations! You've successfully learned how to send personalized emails using Python. From simple greetings to handling attachments, this guide has equipped you with the knowledge to create engaging content for your recipients. Feel free to explore more advanced features and customize the script to suit your specific needs.
Remember, personalization goes a long way in creating meaningful connections. Use your newfound skills to make your emails stand out and leave a lasting impression. Happy coding!
0 Comments