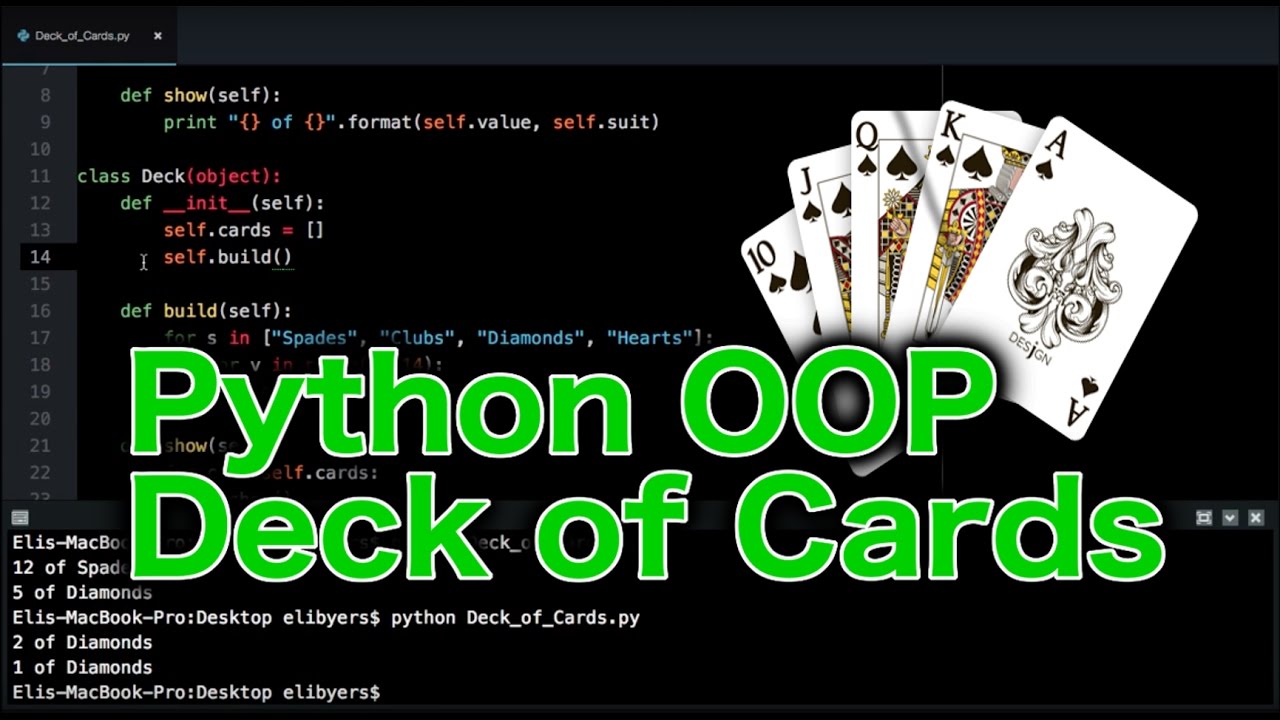
As I delve into the world of building playing card games using Python, I am immediately captivated by the endless possibilities that lie ahead. The idea of combining my passion for Python programming with the excitement of card games sparks a sense of curiosity and anticipation. The introduction promises a journey through the creation of a deck of cards, shuffling techniques, dealing strategies, and even the opportunity to construct a simple card game from scratch. The comprehensive table of contents ensures that I will navigate through the tutorial effortlessly, allowing me to absorb each step with ease. With the potential to develop more complex games and add personalized features, I am eager to embark on this thrilling adventure of coding and entertainment.
Table of Contents
- Introduction
- Creating a Deck of Cards
- Shuffling the Deck
- Dealing the Cards
- Building a Card Game
- Conclusion
Introduction
Playing card games have always been a popular pastime, whether it's a friendly game of poker or a strategic battle of blackjack. If you're a Python enthusiast and a fan of card games, you can combine your passions by building your very own card games using the power of Python programming language. In this tutorial, we will explore how to create a deck of cards, shuffle them, deal them to players, and even build a simple card game from scratch.
Creating a Deck of Cards
Before we can start playing card games, we need a deck of cards. A deck consists of 52 cards, divided into four suits (hearts, diamonds, clubs, and spades) and thirteen ranks (Ace, 2-10, Jack, Queen, and King). In Python, we can represent a deck of cards using a combination of lists and loops. We'll define the suits and ranks as lists and use nested loops to create the complete deck.
import itertools
suits = ['Hearts', 'Diamonds', 'Clubs', 'Spades']
ranks = ['Ace', '2', '3', '4', '5', '6', '7', '8', '9', '10', 'Jack', 'Queen', 'King']
deck = list(itertools.product(ranks, suits))
Shuffling the Deck
Now that we have a deck of cards, it's important to shuffle them to ensure fairness and randomness in the game. Python provides the random
module, which contains functions for generating random numbers. We can use the random.shuffle()
function to shuffle the deck of cards.
import random
random.shuffle(deck)
Dealing the Cards
Once the deck is shuffled, we can deal the cards to the players. The number of cards each player receives depends on the game being played. In Python, we can represent the players as lists and use list slicing to distribute the cards evenly among them.
num_players = 4
hand_size = len(deck) // num_players
players = [deck[i * hand_size:(i + 1) * hand_size] for i in range(num_players)]
Building a Card Game
Now that we have a deck of cards and the ability to shuffle and deal them, we can build a simple card game. Let's take the example of a simplified version of the popular game "War," where two players compete by comparing the ranks of their cards. The player with the higher rank wins the round.
player1 = players[0]
player2 = players[1]
while len(player1) > 0 and len(player2) > 0:
card1 = player1.pop(0)
card2 = player2.pop(0)
rank1 = ranks.index(card1[0])
rank2 = ranks.index(card2[0])
if rank1 > rank2:
player1.extend([card1, card2])
elif rank2 > rank1:
player2.extend([card1, card2])
else:
player1.append(card1)
player2.append(card2)
if len(player1) > 0:
print("Player 1 wins!")
else:
print("Player 2 wins!")
Conclusion
By using the power of Python programming language, you can build your own card games and explore the endless possibilities of game development. In this tutorial, we learned how to create a deck of cards, shuffle them, deal them to players, and even build a simple card game from scratch. With this knowledge, you can now dive deeper into more complex card games or add additional features to your existing games. So, grab your deck of cards and start coding your way to a world of endless fun and entertainment!
0 Comments